Deutsche Telekom Developer Center
Communication APIs
Integrate sms, voice, video and two-factor authentication into your apps with Vonage communication APIs. Build complex conversational flows with a user friendly drag-and-drop interface in Vonage AI Studio.
Add an extra layer of security when users perform sensitive tasks by confirming their identities.

Generate a 1-to-1 video appointment workflow. This can be used for a doctor-patient, student-teacher, or any other 1-to-1 web scheduling application.
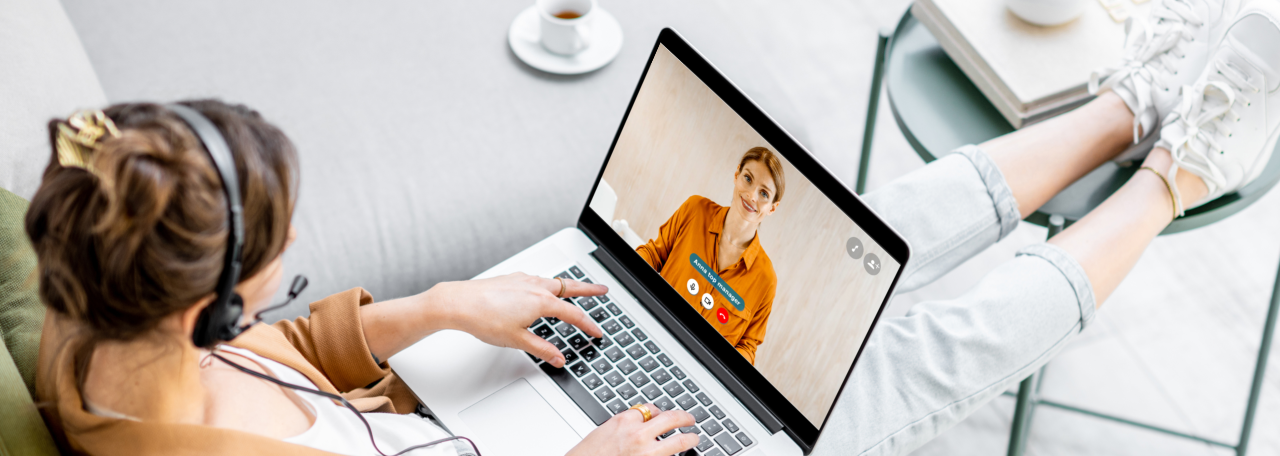
Build an automated phone system for users to input information with the keypad and hear a spoken response

Facilitate SMS communication between two parties without revealing either one's real phone number to the other.
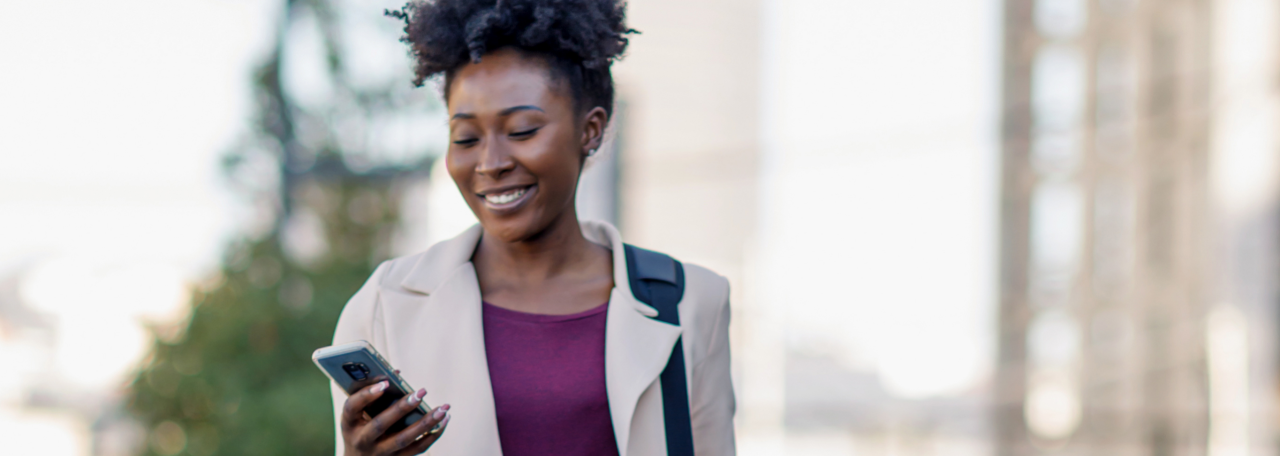
Build an automated phone system for users to input information with the keypad and hear a spoken response

Replace your toll-free numbers (e.g. 800, 0800) with local geo numbers for cheaper local calls and location-sensitive information.
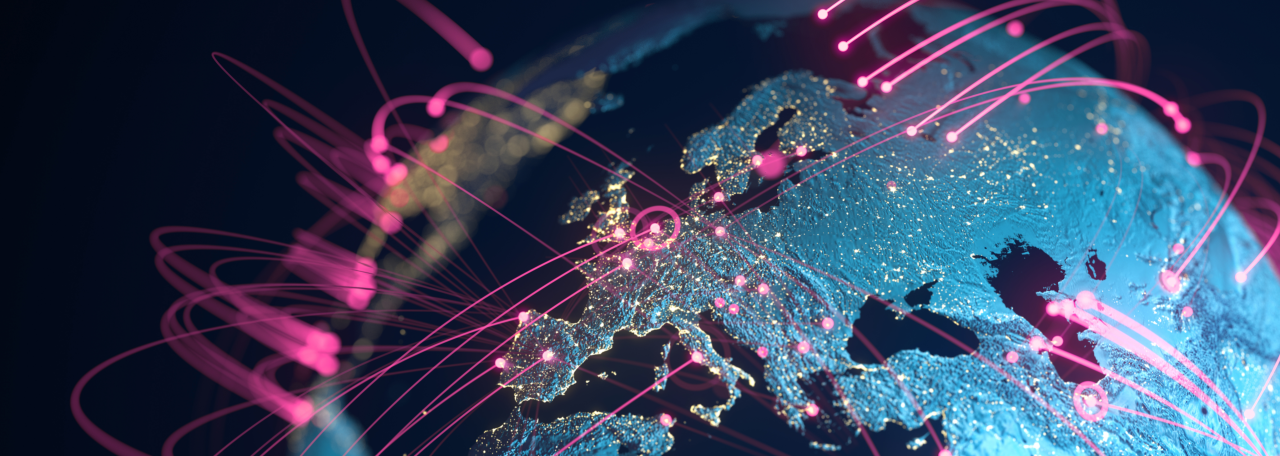
Learn how to enable your customers to call you directly from your website.

Generate a 1-to-1 video appointment workflow. This can be used for a doctor-patient, student-teacher, or any other 1-to-1 web scheduling application.
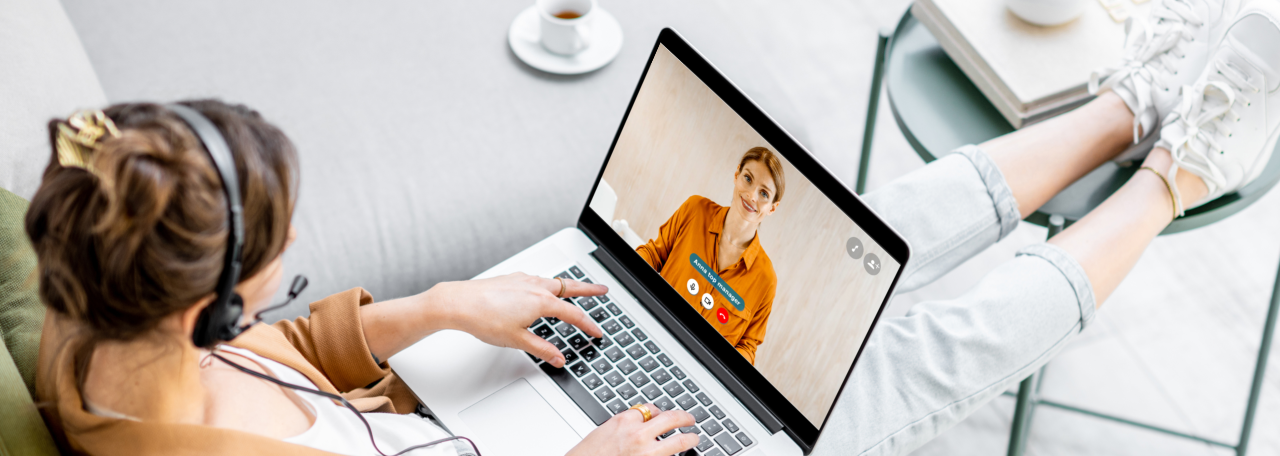
Build your own contact center application.
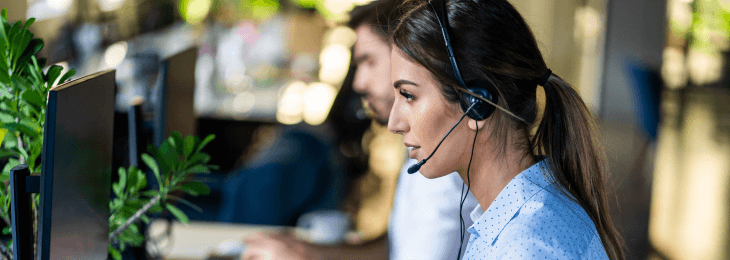
Use the Number Insight and Developer API from Ruby code to validate, sanitize and determine the cost to call or message a number.
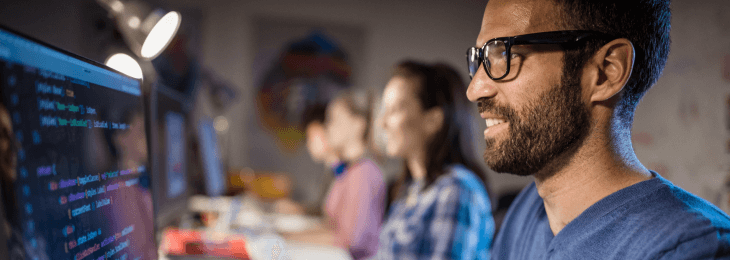
Add an extra layer of security when users perform sensitive tasks by confirming their identities.
