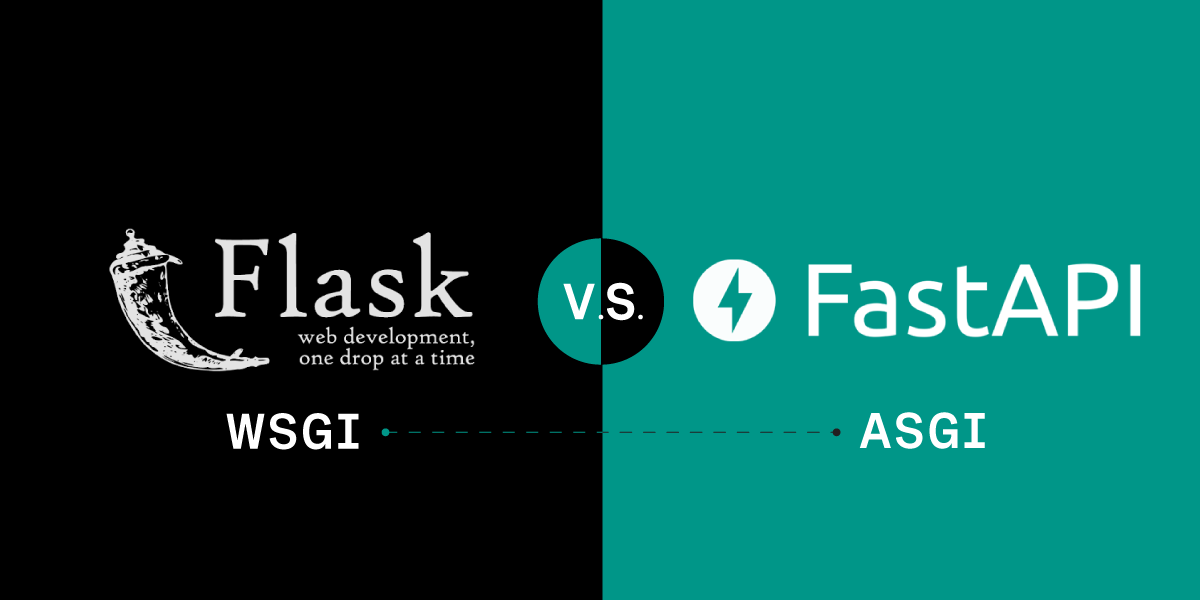
This article was updated by Diana Pham in April 2025
Introduction
If you've ever been confused about the difference between WSGI and ASGI, you're not alone! Let's break it down using a simple (and delicious) analogy: baking a cake.
What Are WSGI and ASGI?
WSGI (Web Server Gateway Interface) and ASGI (Asynchronous Server Gateway Interface) define how a Python web server communicates with web applications. They sit between the web server and your Python framework, handling incoming requests. However, they handle requests in fundamentally different ways.
WSGI v. ASG Iinterface
WSGI: The Traditional, Synchronous Approach
WSGI processes requests synchronously, meaning each request is handled one at a time, in order. The next request must wait for the previous one to finish before it can be processed.
If you've used Flask, you've encountered WSGI—it’s been the Python web standard for years. However, this sequential processing can slow things down, especially when handling many requests simultaneously.
ASGI: The Modern, Asynchronous Approach
ASGI, the successor to WSGI, introduces asynchronous request handling. This means multiple requests can be processed at the same time without waiting on each other.
You may have heard of the new Python web framework, FastAPI. By default, it uses ASGI, which makes it lightning-fast. FastAPI is also a micro web framework with many advantages, including out-of-the-box support for asynchronous code using the Python async and await keywords!
WSGI vs. ASGI: Baking a Cake Analogy
To understand the difference between WSGI and ASGI, imagine you're preparing a birthday party treat: baking a cake and making the frosting from scratch. These are two separate tasks—or in web terms, two incoming requests.
WSGI: One Task at a Time (Synchronous Processing)
With WSGI, each request is handled one after the other. You must complete the first task entirely before moving on to the next.
Request 1: Bake Cake
1. Mix the batter
2. Pour it into pans
3. Bake in the oven (30 min wait)
Request 2: Make Frosting
4. Mix butter and powdered sugar
5. Add vanilla and milk
6. Stir until smooth
So, if baking takes 30 minutes, you’ll be stuck waiting before you can even start the frosting. This is synchronous processing—no overlapping of tasks, even if you're just waiting around.
Synchronous requests
ASGI: Multitasking Between Requests (Asynchronous Processing)
With ASGI, you can handle multiple requests at once. If one is waiting (like the cake in the oven), you can switch over and work on another.
Request 1: Bake Cake
1. Mix the batter
2. Pour it into pans
3. Put it in the oven (starts baking)
→ Switch to Request 2 while the cake bakes…
Request 2: Make Frosting
4. Mix butter and powdered sugar
5. Add vanilla and milk
6. Stir until smooth
→ Return to Request 1 once the cake is done
Instead of standing idle, you're making better use of your time. ASGI works this way—by allowing tasks to pause and resume, handling I/O or other long operations without blocking everything else.
⚠ Note: Not all tasks can run at the same time—some depend on others being completed first. For example, you can’t bake a cake before mixing the batter! Similarly, in ASGI applications, certain operations (like a database query or file read) may still require ordered execution.
Asynchronous requestsPython Pseudocode Examples
WSGI-style Synchronous Code
def bake_cake(request):
mix_batter()
pour_into_pan()
bake_in_oven() # This blocks for a long time
return response
def make_frosting(request):
mix_ingredients()
stir_until_smooth()
return response
Here, make_frosting()
won’t even start until bake_cake()
finishes. Requests are processed one at a time.
ASGI-style Synchronous Code
async def bake_cake(request):
await mix_batter()
await pour_into_pan()
await bake_in_oven() # Can yield control here
return response
async def make_frosting(request):
await mix_ingredients()
await stir_until_smooth()
return response
In this async version, while bake_in_oven()
is waiting, the server can jump over and run make_frosting()
instead of idling. That’s the power of ASGI—non-blocking, efficient multitasking.
Boxing It All Up
WSGI is like baking a cake and standing still while it’s in the oven. ASGI lets you make the frosting in the meantime. Both have their use cases, but if you need scalable, high-performance web apps that can handle many tasks efficiently, ASGI is your go-to.
Got any questions or comments? Join our thriving Developer Community on Slack, follow us on X (formerly Twitter), or subscribe to our Developer Newsletter. Stay connected, share your progress, and keep up with the latest developer news, tips, and events!